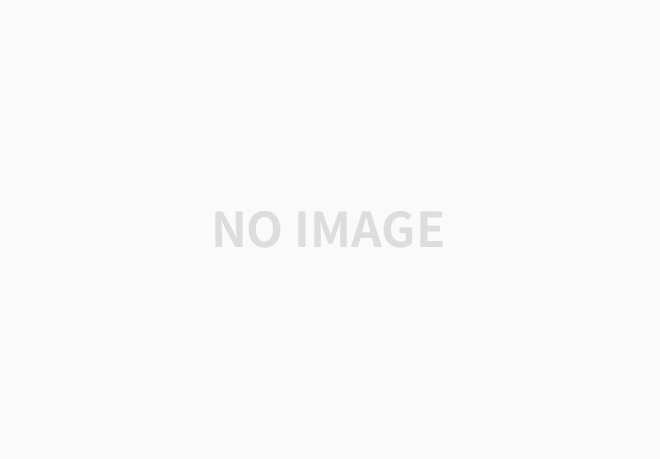
프로토타입 패턴이란?
생산 비용이 높은 인스턴스를 복사를 함으로써 쉽게 생성할 수 있도록 도와주는 패턴
실습
일러스트레이터와 같이 그림 그리기 툴을 개발 중이다.
어떤 모양을 그릴 수 있도록 하고 복사 붙여 넣기 기능을 구현해보자
public class Shape implements Cloneable{
private String id;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
public class Circle extends Shape{
private int x;
private int y;
private int r;
public Circle(int x, int y, int r) {
this.x = x;
this.y = y;
this.r = r;
}
@Override
public String toString() {
return "Circle{" +
"x=" + x +
", y=" + y +
", r=" + r +
'}';
}
public Circle copy() throws CloneNotSupportedException {
Circle clone = (Circle) clone();
clone.x = x + 1;
clone.y = y + 1;
return clone;
}
}
public class Main {
public static void main(String[] args) throws CloneNotSupportedException {
Circle circle1 = new Circle(1,1,3);
Circle circle2 = circle1.copy();
System.out.println(circle1.toString());
System.out.println(circle2.toString());
}
}
Object 클래스의 clone을 재정의 하는 클래스를 생성하여 (Shape)
해당 클래스를 상속 받음으로써 객체에 대한 복사를 가능하게 한다.
'CS > DesignPattern' 카테고리의 다른 글
(DesignPattern) 추상팩토리 메서드 패턴 (0) | 2020.05.16 |
---|---|
(DesignPattern) 빌더 패턴 (0) | 2020.05.10 |
(DesignPattern) 싱글턴 패턴 (0) | 2020.05.02 |
(DesignPattern) 팩토리메서드 패턴 (0) | 2020.04.22 |
(DesignPattern) 어댑터 패턴 (0) | 2020.04.21 |