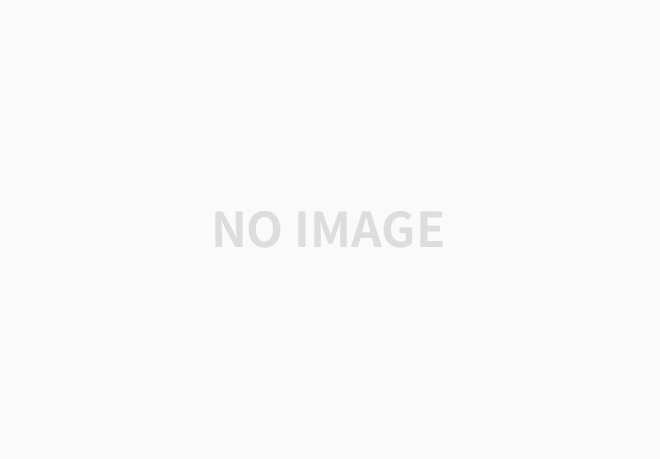
선택 정렬이란?
가장 작은 요소부터 선택해 알맞은 위치로 옮겨서 순서대로 정렬하는 알고리즘
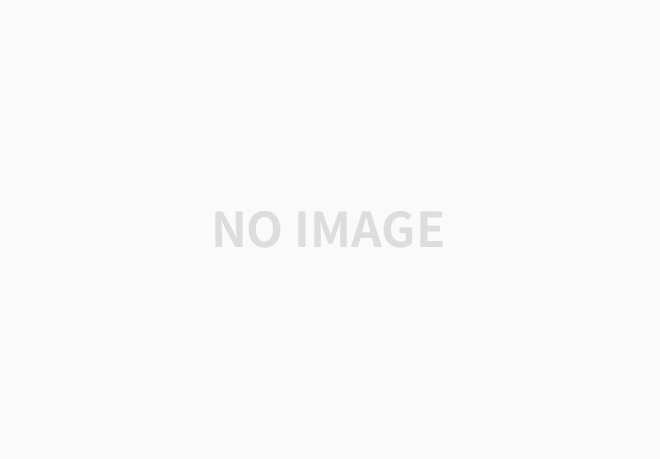
해당 배열에서 가장 작은 값 : 1
1과 6을 스위칭
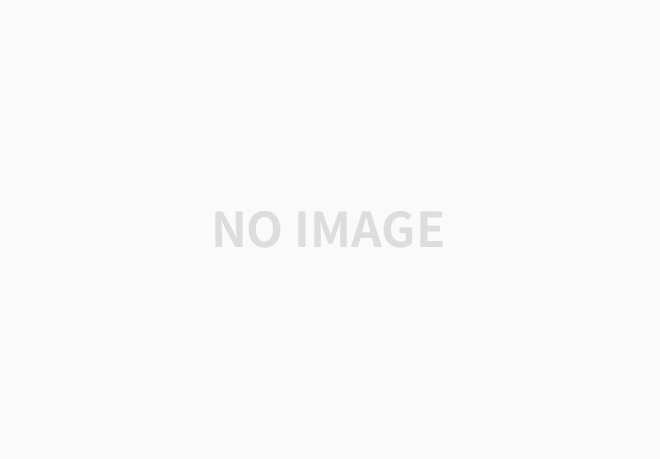
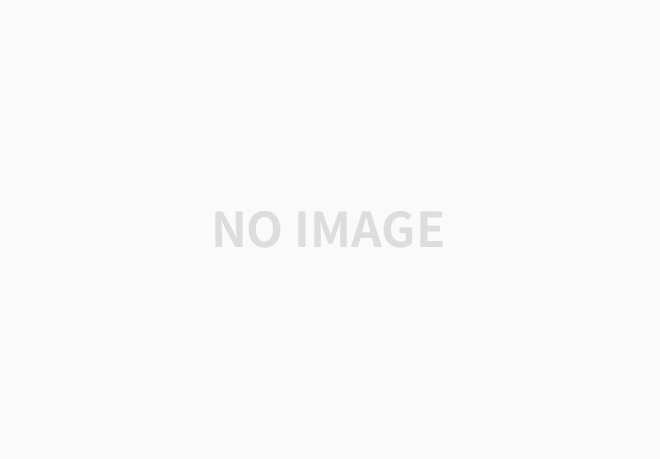
그다음 작은 값 : 3
4와 3을 스위칭
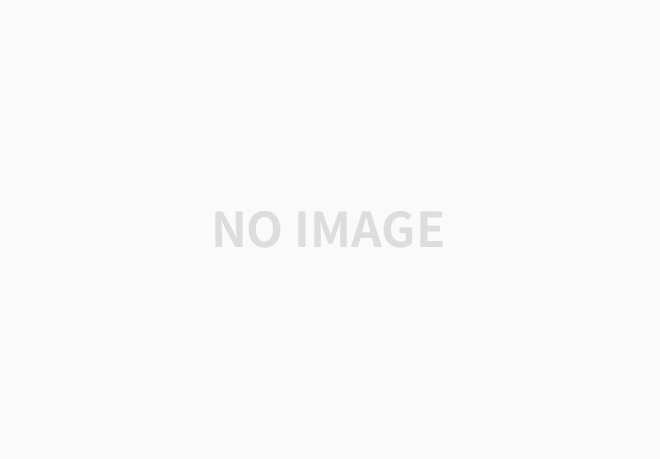
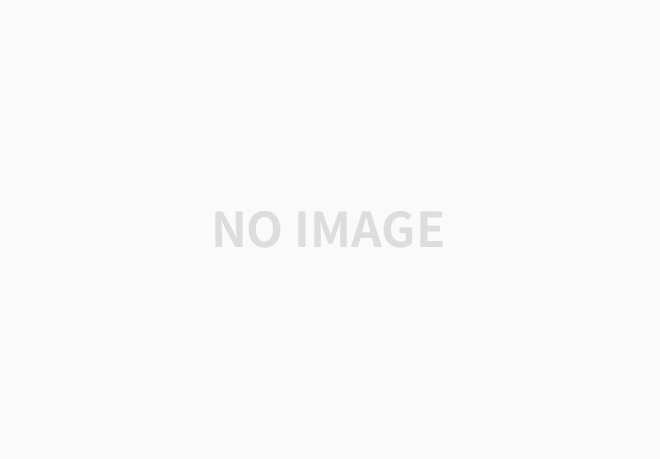
이와 같은 과정을 반복하면 오름차순으로 정렬이 완료된다.
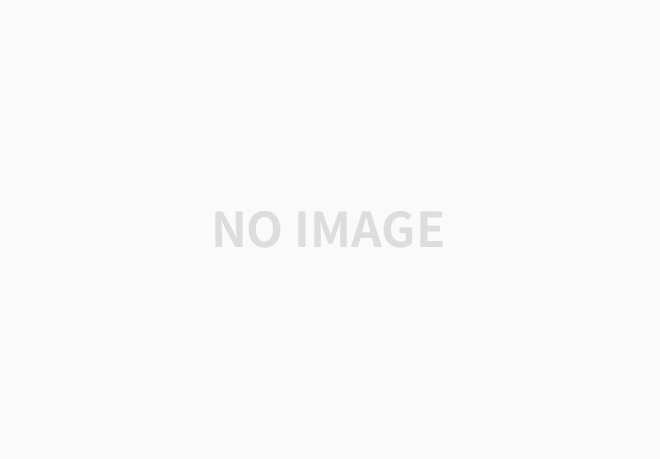
구현
public class SelectionSort {
// static int min = Integer.MAX_VALUE;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("선택정렬");
System.out.println("요솟수 : ");
int nx = sc.nextInt();
int[] x = new int[nx];
for (int i=0 ;i<nx; i++) {
x[i] = sc.nextInt();
}
selectionSort(x, nx);
for (int i=0 ;i<nx; i++) {
System.out.printf("x["+i+"]="+x[i]+ " ");
}
}
private static void selectionSort(int[] array, int length) {
//6 4 8 3 1 9 7
for (int i=0 ; i<length ; i++) {
// int min = Integer.MAX_VALUE;
int minIndex = i;
for (int k=i ; k<length ; k++) {
if (array[k] < array[minIndex])
minIndex= k;
// if (min > array[k]) {
// min = array[k];
// minIndex = k;
// System.out.println("minValue :: "+min);
// System.out.println(minIndex);
// }
}
swap (minIndex, i, array);
}
}
static void swap (int minIndex, int startIndex, int[] array) {
int temp = array[minIndex];
array[minIndex] = array[startIndex];
array[startIndex] = temp;
}
}
Code Link
https://github.com/mike6321/PURE_JAVA/tree/master/Algorithm
mike6321/PURE_JAVA
Contribute to mike6321/PURE_JAVA development by creating an account on GitHub.
github.com
'CS > Algorithm' 카테고리의 다른 글
(알고리즘) N과 M (2) - 조합 (0) | 2020.10.21 |
---|---|
(알고리즘) N과 M (1) - 순열 (0) | 2020.10.20 |
(알고리즘) 순열과 조합의 차이 (0) | 2020.10.20 |
(알고리즘) 정렬 (3) - 퀵정렬 (0) | 2020.02.26 |
(알고리즘) 정렬 (1) - 개요 및 버블 정렬 (0) | 2020.02.23 |